Running Cross-Browser Testing With Jest
by Abdul Aziz Mondal Information Technology 22 August 2023
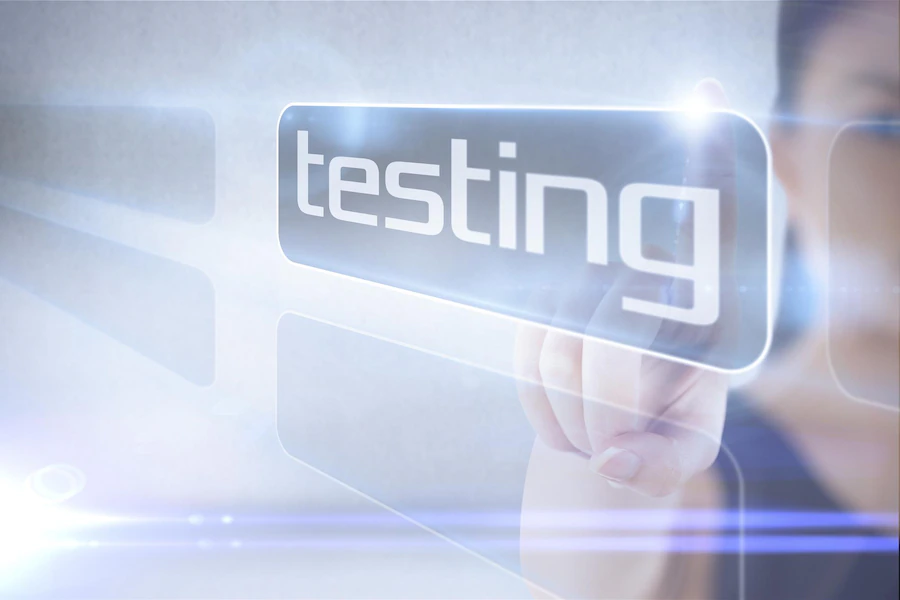
In the constantly growing field of web development, presenting a clean and steady consumer experience through a number of browsers is crucial. Cross-browser testing has grown in relevance as customers get entry to websites and online applications using of various devices and browsers. Differences in rendering engines, JavaScript execution, and CSS parsing among browsers can lead to layout inconsistencies, functionality issues, and performance discrepancies. To address these challenges, developers focus on powerful testing frameworks like Jest to conduct cross-browser testing effectively.
The Significance Of Cross-Browser Testing
With so many mainstream browsers, web development has become increasingly difficult. On PCs, laptops, tablets and smartphones, people can access websites and packages through various browsers such as Google Chrome, Apple Safari, Microsoft Edge, etc. Each browser interprets web content differently, leading to visual and functional discrepancies across platforms. Cross-browser testing ensures that a website’s functionality remains consistent and visually appealing across various browser environments, guaranteeing an optimal user experience for all visitors.
Leveraging The Power Of Jest For Cross-Browser Testing
1. Rich Feature Set: Jest has gained widespread popularity in the JavaScript community due to its rich feature set. From snapshot testing, code coverage analysis, and mocking to parallel test execution and easy-to-read test output, Jest provides a comprehensive toolkit for writing and executing test cases.
2. Easy Setup: Setting up Jest for cross-browser testing is straightforward. Developers may instantly get started creating tests using a user-friendly UI and a minimal configuration approach, allowing them to focus on ensuring the robustness of their software.
3. Community Support and Popularity:
As a Facebook product, Jest has gained tremendous popularity amongst developers, resulting in an active community that contributes to its growing development. Jest is a dependable solution for cross-browser testing due to the extensive community support that ensures regular updates, bug fixes and the availability of various online resources and plugins.
Consider using LambdaTest for a really smooth and efficient cross-browser testing experience. LambdaTest reduces the complexities of testing across multiple contexts with its cloud-based infrastructure, with 3000+ browsers and real device cloud for compatibility and a user-friendly interface. This integrated approach, together with the techniques discussed in this article, enables developers to create web apps that consistently please consumers across several browsers and devices, resulting in a superior user experience.
Preparing The Project For Cross-Browser Testing
1. Installing Jest And Necessary Dependencies:
To start cross-browser testing with Jest, make sure you have Node.Js installed on your system. Create a new project in the terminal or use an existing one. Configure Jest and its dependencies using the following instructions.
npm init -y
npm install jest puppeteer jest-environment-jsdom-global –save-dev
2. Configuring Jest:
To configure Jest for cross-browser testing, create a `jest.config.js` file in your project with the following information.
javascript
module.export={
testEnvironment: “jest-environment-jsdom-global”,
testMatch: [“**/__tests__/**/*.js?(x)”, “**/?(*.)+(spec|test).js?(x)”],
};
The `testEnvironment` setting ensures that Jest runs tests using jsdom, an in-memory DOM implementation. The `testMatch` setting specifies the patterns for Jest to look for test files.
Writing Cross-Browser Test Suites
1. Creating Test Files:
To effectively organize your tests, create separate files or directories for different components or features. For example, you can set up a `__tests__` directory at the root of your project to store all test files. Jest will automatically discover and execute these test files.
2. Writing Test Cases:
Each test file should contain one or more test cases, represented by functions using the `test` keyword. A test case typically follows the structure of “Arrange, Act, Assert”:
– Arrange: Set up the test environment, including any necessary data and configurations.
– Act: Execute the code or functionality you want to test.
– Assert: Verify that the output matches the expected result.
Example:
javascript
// sum.js
function sum(a, b) {
return a + b;
}
module.exports = sum;
javascript
// sum.test.js
const sum = require(“./sum”);
test(“adds 1 + 2 to equal 3”, () => {
expect(sum(1, 2)).toBe(3);
});
Executing Cross-Browser Tests
1. Running Tests Locally:
To run Jest tests locally, execute the following command in the terminal
npx jest
Jest will automatically discover and execute the test files in the `__tests__` directory and display the results in the terminal.
2. Integrating with Continuous Integration (CI) Tools:
To ensure cross-browser tests are automatically executed whenever changes are pushed to the repository, integrate Jest with popular CI tools like Jenkins, Travis CI, or GitHub Actions. This step ensures that your codebase remains robust and free of cross-browser issues throughout the development process.
Running Cross-Browser Tests With Puppeteer
Puppeteer, developed by Google, is a powerful library that allows developers to control headless or full browsers programmatically. By integrating Puppeteer with Jest, developers can run cross-browser tests on real browser instances, enabling the detection of issues that may not be apparent in the jsdom environment.
1. Installing Puppeteer:
Puppeteer may be used for cross-browser testing by using the following command to install it as a development dependency:
npm install puppeteer- save-dev
2. Modifying Test Configuration:
To utilize Puppeteer as the test environment, modify the ‘jest.config.js’ folder as follows
javascript
module.exports={
testEnvironment: “jest-environment-jsdom-global”,
testMatch: [“**/__tests__/**/*.js?(x)”, “**/?(*.)+(spec|test).js?(x)”],
testEnvironmentOptions: {
puppeteer: {
headless: true, // Set to false to run tests in a visible browser window
},
},
};
3. Writing Puppeteer Test Cases:
With Puppeteer integrated, developers can write test cases that control real browser instances. Puppeteer offers a range of methods to interact with the browser, including navigating to pages, clicking elements, and evaluating JavaScript code.
Example:
javascript
// example.test.js
const puppeteer = require(“puppeteer”);
test(“must browse to the homepage”, async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto(“https://www.example.com”);
expect(await page.title()).toBe(“Example Domain”);
await browser.close();
});
Dealing With Cross-Browser Compatibility Issues
1. Identifying Browser-Specific Bugs:
When cross-browser tests fail, carefully analyze the issues and their relation to specific browsers. Use browser developer tools to inspect and debug problematic elements or JavaScript code.
2. Implementing Browser-Specific Solutions:
If you encounter browser-specific bugs, consider using feature detection, polyfills, or conditional code blocks to provide specific solutions for different browsers. Libraries like Modernizr and Polyfill can assist in this process.
Handling Browser Versions And Legacy Support
In addition to testing across various browsers, it is essential to consider different browser versions. Older browser versions may lack support for modern JavaScript features and CSS properties, resulting in inconsistent behavior. Conducting testing on legacy browsers and providing graceful degradation ensures your application remains functional for a broader range of users.
Cross-Browser Testing Best Practices
Follow these best practices to maximize the effectiveness of cross-browser testing using Jest
1. Update dependencies such as Jest and Puppeteer on a regular basis to take advantage of the most recent features and bug patches.
2. Use Jest’s code coverage analysis to find portions of your software that need more testing or restructuring.
3. Configure continuous integration to run tests automatically anytime the repository is changed, enabling early bug detection and better development workflows.
4. Include real-world user scenarios in your test cases to simulate genuine surfing activity and identify potential concerns.
The Future of Cross-Browser Testing
Cross-browser testing will remain an important technique for web developers as web technologies improve. Jest and other testing frameworks can be expected to keep up with developing browser technology, providing the essential tools and support for complete cross-browser testing.
Automation And Scalability In Cross-Browser Testing
Manual cross-browser testing becomes impractical and time-consuming when web development projects grow in complexity and breadth. Automation and scalability are critical in expediting the testing process in order to meet this difficulty. Jest’s compatibility with various testing environments and Puppeteer’s ability to run tests on multiple browser instances enable developers to automate cross-browser testing efficiently.
1. Leveraging Jest’s Test Environment Options:
Jest’s flexibility allows developers to configure different test environments based on their requirements. By customizing the test environment options in the `jest.config.js` file, developers can run tests using real browsers, such as Chrome, Firefox, or headless browsers. This flexibility empowers teams to create custom testing environments that suit their specific cross-browser testing needs.
2. Parallel Test Execution:
One of Jest’s significant advantages is its ability to execute tests in parallel, which significantly reduces the overall testing time. By spreading tests across multiple CPU cores, Jest optimizes the test execution process, making cross-browser testing faster and more efficient. This parallelization is especially beneficial when running extensive test suites that encompass numerous browsers and devices.
3. Integrating With Cloud-Based Testing Services:
Due to technology constraints and the necessity for a diverse collection of browser configurations, doing cross-browser tests locally may not be feasible for large-scale projects. Cloud-based testing services such as Sauce Labs and BrowserStack provide virtual testing environments with a wide range of browsers and operating systems. Integrating Jest and Puppeteer with these services allows developers to perform cross-browser testing across various real devices and browsers simultaneously, enhancing test coverage and scalability.
The Role Of Code Coverage Analysis In Cross-Browser Testing
Code coverage analysis is a crucial aspect of any testing process, including cross-browser testing. Jest’s built-in code coverage feature provides valuable insights into the parts of the codebase that the tests cover. By analyzing code coverage reports, developers can identify areas that require more extensive testing, ensuring better cross-browser compatibility. This analysis helps in detecting browser-specific issues and potential gaps in test coverage, enhancing the overall quality of the application.
(CI/CD) Integration: Continuous Integration And Continuous Deployment
Developers widely use these methods to ensure that cross-browser testing is seamlessly integrated into the development workflow. CI/CD pipelines automatically trigger tests whenever changes are pushed to the version control repository. By integrating Jest and Puppeteer with CI/CD tools like Jenkins, Travis CI, or GitHub Actions, developers can detect and address cross-browser compatibility issues early in the development process. The automation provided by CI/CD pipelines ensures that every code change is thoroughly tested across various browsers and devices before being deployed to production.
Test Maintenance And Regression Testing
As a web application evolves, so do the cross-browser testing requirements. New features, bug fixes, and browser updates can impact existing functionality. Therefore, maintaining and updating test suites is essential. Regularly reviewing and updating tests to accommodate changes in the application’s codebase and browser landscape ensures that cross-browser testing remains effective and relevant over time.
Cross-browser testing with Jest and Puppeteer offers web developers a powerful and comprehensive approach to ensure their applications function seamlessly across various browsers and devices. The combination of Jest’s rich feature set, easy setup, and parallel test execution, along with Puppeteer’s ability to run tests on real browsers, empowers developers to uncover browser-specific issues efficiently. Automation and scalability further enhance the cross-browser testing process, enabling teams to test extensively and swiftly, regardless of the project’s size or complexity.
Read Also: